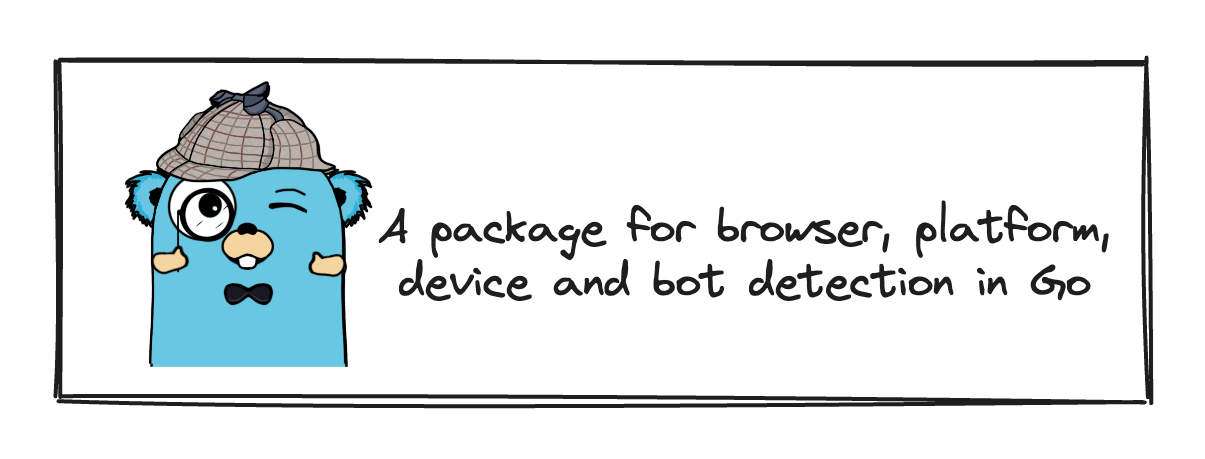
Go Browser Package #
I have been working on a project that required me to identify the browser, device, platform, or bot making a request to my application. The URL shortening service needed to generate analytics and render Open Graph (OG) tags that were optimized for the client.
I wanted to build the service in Go, a fast and efficient language well-suited for building web applications. However, I could not find a simple and easy-to-use package, so I decided to build the Browser package to fill this gap.
Why use the Browser package? #
The Browser package is a simple, standalone tool for parsing user agents. It integrates easily into your application without adding overhead and is designed for speed and efficiency.
Accurate and reliable #
The regex-based matchers provided by the Browser package are highly accurate and can identify a wide range of browsers, devices, platforms, and bots. The package is regularly updated to ensure it is up-to-date with the latest browser, device, platform, and bot information.
Tested against commercial user-agent database #
The regexes are tested against our 250 million user-agent database, which I bought from a company. This ensures the regexes are accurate and reliable and helps me improve them and add new ones as needed.
Usecases #
The Browser package has a wide range of use cases, including:
- Analytics: Identify browsers, devices, platforms, or bots making requests to generate detailed analytics reports.
- Content delivery: Optimize content delivery based on the user agent.
- SEO: Improve your search engine optimization (SEO) by delivering optimized content to search engine bots for their requirements.
- OG Tags: Generate Open Graph (OG) tags optimized for the browser, device, platform, or bot requesting your application.
- User experience: Improve user experience by delivering tailored content.
- Security: Identify and block malicious bots making requests to your application.
Quick Start #
To install the Browser package, you can use the below command:
go get github.com/dineshgowda24/browser
Import the package into your application and create a new instance of the Browser
struct by passing the user agent string to the NewBrowser
function.
package main
import (
"fmt"
"github.com/dineshgowda24/browser"
)
func main() {
b, err := browser.NewBrowser("Mozilla/5.0 (Linux; Android 10; SM-A205U) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/90.0.4430.210 Mobile Safari/537.36")
if err != nil {
// handle error
}
}
Matchers #
Matchers identify the browser making a request to your application. The package currently supports a wide range of matchers that can identify most of the popular browsers in use today.
Usage #
To use the matchers, you can create a new instance of the Browser
struct.
b, err := browser.NewBrowser("Mozilla/5.0 (Linux; Android 10; SM-A205U) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/90.0.4430.210 Mobile Safari/537.36")
if err != nil {
// handle error
}
// browser level information
fmt.Println(b.Name()) // Chrome
fmt.Println(b.Version()) // 90.0.4430.210
fmt.Println(b.ShortVersion()) // 90
fmt.Println(b.IsBrowserKnown()) // true
fmt.Println(b.IsChrome()) // true
What if the browser is not supported? #
The matchers can be extended by adding a custom regex to the package. This allows you to identify browsers not supported by the package out of the box.
The custom matcher should implement the below interface:
type Matchers interface {
Match() bool // Match returns true if the user agent string matches the matcher.
Name() string // Name returns the name of the matcher.
}
type BrowserMatcher interface {
Matcher
Version() string // Version returns the full version of the browser.
}
You can raise an issue or create a pull request to add the custom browser to the package. If it meets the requirements, I will be happy to look at it and merge it.
Supported Matchers #
The package currently supports the following matchers:
- Nokia
- Alipay
- UCBrowser
- BlackBerry
- Brave
- Opera
- Otter
- Snapchat
- MicroMessenger
- Electron
- DuckDuckGo
- GoogleSearchApp
- HuaweiBrowser
- Konqueror
- Maxthon
- MiuiBrowser
- PaleMoon
- Puffin
- Edge
- InternetExplorer
- SamsungBrowser
- SogouBrowser
- Vivaldi
- VivoBrowser
- Sputnik
- YaaniBrowser
- Yandex
- Firefox
- Chrome
- Safari
Platforms #
Platforms are used to identify the platform that is making a request to your application. Examples of platforms include Windows, macOS, Linux, iOS, and Android.
Usage #
To use the platforms, you can create a new instance of the Browser
struct and call it the Platform
method. If the user agent string matches any of the platforms, the Platform
method will return the name of the platform.
b, err := browser.NewBrowser("Mozilla/5.0 (Linux; Android 10; SM-A205U) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/90.0.4430.210 Mobile Safari/537.36")
if err != nil {
// handle error
}
// platform level information
fmt.Println(b.Platform().Name()) // Android
fmt.Println(b.Platform().Version()) // 10
fmt.Println(b.Platform().IsAndroidApp()) // false
What if the platform is not supported? #
If the platform is not supported by the package out of the box, you can add custom platforms by implementing the below interface:
type Matchers interface {
Match() bool // Match returns true if the user agent string matches the matcher.
Name() string // Name returns the name of the matcher.
}
type PlatformMatcher interface {
Matcher
Version() string // Version returns the version of the platform.
}
You can raise an issue or create a pull request to add the custom platform to the package. I will happily look at it and merge it if it meets the requirements.
Supported Platforms #
The package currently supports the following platforms:
- AdobeAir
- BlackBerry
- KaiOS
- IOS
- WatchOS
- WindowsMobile
- WindowsPhone
- Windows
- Android
- Linux
- FirefoxOS
- ChromeOS
Devices #
Devices are used to identify the device that is making a request to your application. Examples of devices include desktops, laptops, tablets, and smartphones.
Usage #
To use the devices, you can create a new instance of the Browser
struct and call it the Device
method. If the user agent string matches any of the devices, the Device
method will return the name of the device.
b, err := browser.NewBrowser("Mozilla/5.0 (Linux; Android 10; SM-A205U) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/90.0.4430.210 Mobile Safari/537.36")
if err != nil {
// handle error
}
// device level information
fmt.Println(b.Device().Name()) // Samsung SM-A205U
fmt.Println(b.Device().IsTablet()) // false
fmt.Println(b.Device().IsSamsung()) // true
What if the device is not supported? #
If the device is not supported by the package out of the box, you can add custom devices by implementing the below interface:
type Matchers interface {
Match() bool // Match returns true if the user agent string matches the matcher.
Name() string // Name returns the name of the matcher.
}
type DeviceMatcher interface {
Matcher
}
You can raise an issue or create a pull request to add the custom browser to the package. I will happily look at it and merge it if it meets the requirements.
Supported Devices #
- BlackberryPlaybook
- Iphone
- Ipad
- IpodTouch
- KindleFire
- Kindle
- PlayStation3
- PlayStation4
- PlayStation5
- PSVita
- PSP
- WiiU
- Wii
- XboxOne
- Xbox360
- Switch
- Surface
- Kindle
- Samsung
- TV
- Android
Bots #
Bots are used to identify the bot that is making a request to your application. Examples of bots include Googlebot, Bingbot, and Yandexbot.
Usage #
To use the bots, you can create a new instance of the Browser
struct and call it the Bot
method. If the user agent string matches any bots, the Bot
method will return the bot’s name.
b, err := browser.NewBrowser("Mozilla/5.0 (compatible; Googlebot/2.1; +http://www.google.com/bot.html)")
if err != nil {
// handle error
}
// browser level information
fmt.Println(b.Name()) // Unknown Browser
fmt.Println(b.Version()) // 0.0
fmt.Println(b.ShortVersion()) // 0
fmt.Println(b.IsBrowserKnown()) // false
fmt.Println(b.IsUnknown()) // true
// bot level information
fmt.Println(b.Bot().Name()) // "Googlebot"
fmt.Println(b.Bot().IsBot()) // true
fmt.Println(b.Bot().Why()) // *bots.Known
Supported Bots #
The package supports a large number of bots. To see the complete list of supported bots, please refer to the documentation